Component testing in Cypress allows individual components of an application to be tested in isolation to ensure they function correctly before being integrated into the entire system. This is particularly useful for applications developed with frameworks like React, Angular, or Vue.
What is Component Testing in Cypress?
By testing components, the functionality of small units of code can be verified without being dependent on the overall structure of the system.
This testing approach is especially practical for frontend development, as it enables testing both the visual representation of a component and the underlying logic simultaneously—without having to worry about integration with the backend, REST APIs, or storage systems.
In the typical testing pyramid, which is used to illustrate different testing methods and their scope, component testing is positioned in the middle—between End-to-End (E2E) tests and unit tests.
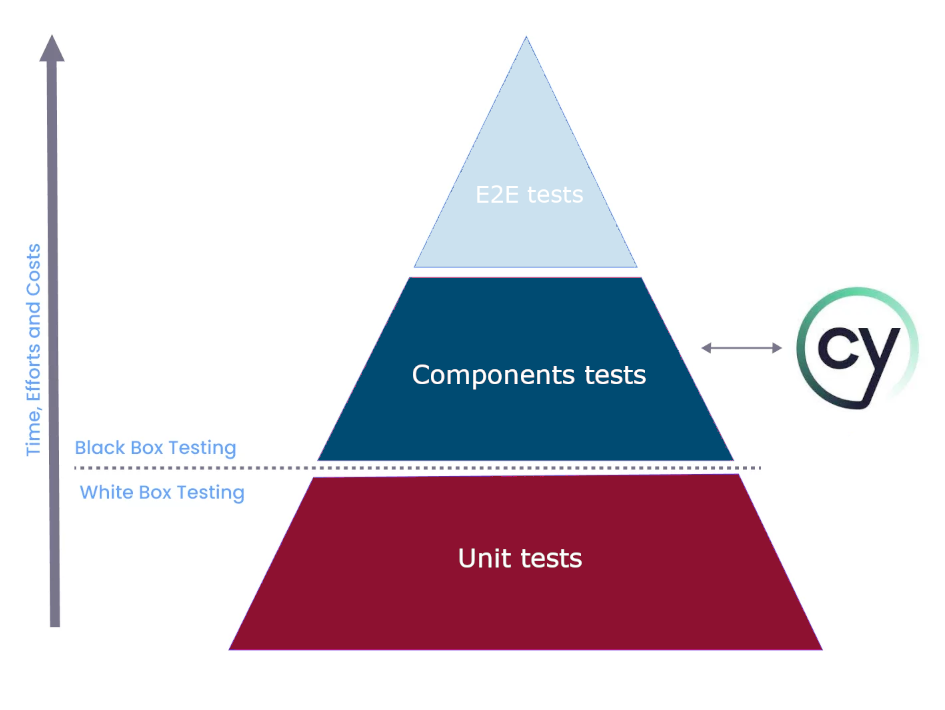
Example of Component Testing in Cypress
A component test in Cypress “mounts” the component by making it independent of any parent page or component to which it originally belonged in the program hierarchy. It isolates the component at runtime, allowing us to perform the necessary assertions.
For example, let’s consider a simple button encapsulated as a component in Angular:
Angular Component (button.component.ts)
import { Component, Input } from '@angular/core';
@Component({
selector: 'app-button',
template: '<button (click)="handleClick()">{{label}}</button>',
styleUrls: ['./button.component.css']
})
export class ButtonComponent {
@Input() label: string = 'Click me';
handleClick() {
console.log('Button clicked');
}
}
The following file demonstrates a component test in Cypress for the same button, where we verify:
a) The button’s label
b) Its action (in this case, a simple console message)
Component Test in Cypress (button.component.cy.ts)
describe('ButtonComponent', () => {
it('renders correctly and handles click events', () => {
cy.mount('<app-button label="Test Button"></app-button>');
cy.get('button').should('contain', 'Test Button'); //<-- comprobamos el nombre
cy.get('button').click();
cy.window().then(win => {. // <-- Comprobamos la acción
cy.spy(win.console, 'log');
cy.get('button').click();
cy.wrap(win.console.log).should('be.calledWith', 'Button clicked');
});
});
});
Additionally, we could add tests to verify that the component correctly handles different labels and states.
describe('ButtonComponent - Additional Tests', () => {
it('should render with a different label', () => {
cy.mount('<app-button label="New Label"></app-button>');
cy.get('button').should('contain', 'New Label');
});
it('should apply different styles based on properties', () => {
cy.mount('<app-button label="Styled Button" class="primary"></app-button>');
cy.get('button').should('have.class', 'primary');
});
});
Component Testing at WATA Factory
At WATA Factory, we have been using component testing in our projects for quite some time, especially in GesA. It has allowed us to accelerate and optimize frontend development.
Conclusion
Component testing with Cypress allows you to test multiple aspects of the frontend simultaneously—including the UI, Ngrx stores, events, and more—without the complexity of setting up, maintaining, and preparing an environment for a full End-to-End (E2E) test.
You can think of it as a small-scale E2E test—less complex but more focused. So don’t hesitate—just as we have done at WATA Factory: Give component testing in Cypress a chance!